Lets Start,
1. Open Android Studio and create a new project and select a blank activity to start with.
2. If you are on the latest version of Android Studio you don't have to add a compiled dependency of Appcombat v7 21 if not then please make sure you add the line below in your gradel build dependencies.
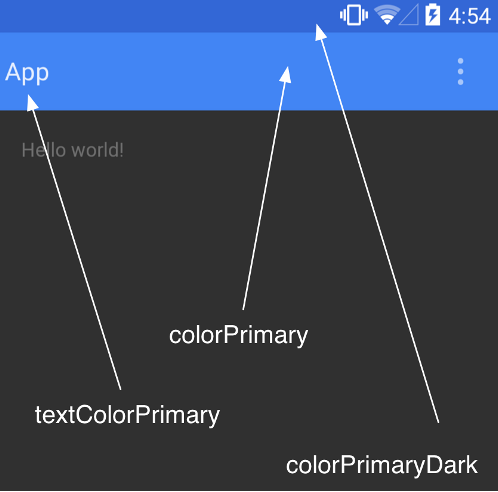
To do that you need make a file called color.xml in your values folder and add the color attributes as shown in the below code.
1
2
3
4
5
6
7
| < span style = "font-size: x-small;" > < resources > < color name = "ColorPrimary" >#FF5722</ color > < color name = "ColorPrimaryDark" >#E64A19</ color > </ resources > </ span > |
And this is how the style.xml looks after adding the colors.
1
2
3
4
5
6
7
8
9
10
11
12
13
| < span style = "font-size: x-small;" >< resources >
< style name = "AppTheme" parent = "Theme.AppCompat.Light.NoActionBar" > < item name = "colorPrimary" >@color/ColorPrimary</ item > < item name = "colorPrimaryDark" >@color/ColorPrimaryDark</ item >
</ style > </ resources > </ span > |
Go to res folder in your project and create a new Layout Resource File and name ittool_bar.xml with the parent layout as android.support.v7.widget.Toolbar as shown in the image below.
6. now add the background color to your tool_bar as the primary color of the app and give an elevation of 4dp for the shadow effect, this is how the tool_bar.xml looks.
1
2
3
4
5
6
7
8
9
10
11
12
| < span style = "font-size: x-small;" > android:layout_width = "match_parent" android:layout_height = "wrap_content" android:background = "@color/ColorPrimary" android:elevation = "4dp" > </ android.support.v7.widget.Toolbar > </ span > |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| < span style = "font-size: x-small;" >< RelativeLayout xmlns:android = "http://schemas.android.com/apk/res/android" android:layout_width = "match_parent" android:layout_height = "match_parent" tools:context = ".MainActivity" > < include android:id = "@+id/tool_bar" layout = "@layout/tool_bar" ></ include > < TextView android:layout_below = "@+id/tool_bar" android:layout_width = "wrap_content" android:layout_height = "wrap_content" android:layout_marginTop = "@dimen/TextDimTop" android:text = "@string/hello_world" /> </ RelativeLayout > </ span > |
At this point this is how the app looks like as below.
8. As you can see, the tool_bar layout is added, but it doesn't quite look like a Action Bar yet, that's because we have to declare the toolBar as the ActionBar in the code, to do that add the following code to the MainActivity.java, I have put comments to help you understand what's going on.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
|
import android.support.v4.widget.DrawerLayout; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.support.v7.app.ActionBarDrawerToggle; import android.support.v7.widget.LinearLayoutManager; import android.support.v7.widget.RecyclerView; import android.support.v7.widget.Toolbar; import android.view.Menu; import android.view.MenuItem; import android.view.MotionEvent; import android.view.View; import android.widget.Toast; public class MainActivity extends ActionBarActivity { /* When using Appcombat support library you need to extend Main Activity to ActionBarActivity. */ private Toolbar toolbar; // Declaring the Toolbar Object @Override protected void onCreate(Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.activity_main); toolbar = (Toolbar) findViewById(R.id.tool_bar); // Attaching the layout to the toolbar object setSupportActionBar(toolbar); // Setting toolbar as the ActionBar with setSupportActionBar() call } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_main, menu); return true ; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true ; } return super .onOptionsItemSelected(item); } }
|
After that, this is how the ToolBar looks
9. Notice that the name of the app "MaterialToolbar" is black as we have set the theme parent to Theme.AppCompat.Light.NoActionBar in step 3 it gives the dark text color, So if you want to set the name as light/white text then you can just add android:theme="@style/ThemeOverlay.AppCompat.Dark" in tool_bar.xml and you would get a light text color for the toolbar text, So finally tool_bar.xml looks like this.
1
2
3
4
5
6
7
8
9
10
11
12
|
android:layout_width= "match_parent" android:layout_height= "wrap_content" android:background= "@color/ColorPrimary" android:theme= "@style/ThemeOverlay.AppCompat.Dark" android:elevation= "4dp" >
|
10. All left is to show you how to add menu items like search icon and user icon, for the icons i use icons4android.com which is free to use and I have also mentioned it in my 5 tools every android developer must know Post. I have downloaded the icons in four sizes as recommended by the official Google design guideline and added them to the drawables folder. You can see the project structure from image below.
11. now I need to add search icon and user icons in the menu_main.xml as shown below
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
tools:context= ".MainActivity" >
android:id= "@+id/action_settings" android:orderInCategory= "100" android:title= "@string/action_settings" app:showAsAction= "never" />
android:id= "@+id/action_search" android:orderInCategory= "200" android:title= "Search" android:icon= "@drawable/ic_search" app:showAsAction= "ifRoom" > |
android:id=
"@+id/action_user"
android:orderInCategory=
"300"
android:title=
"User"
android:icon=
"@drawable/ic_user"
app:showAsAction=
"ifRoom"
>
And now everything is done our App bar looks just how we wanted it to look.
So finally we have done and our App Bar looks just how we had seen above and as we have used the appcombat support library it is compatible on pre lollipop devices as well, hope so you liked the post, if you did please share and comment.
Source : http://www.android4devs.com/2014/12/how-to-make-material-design-app.html
0 comments:
Post a Comment